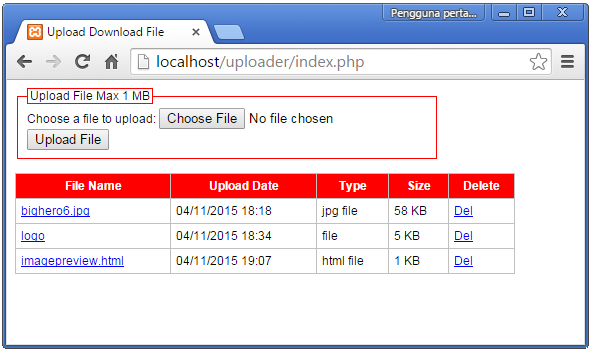
Upload Download File
Pada kesempatan ini saya ingin memberikan coding untuk upload dan download file dengan web browser.
Upload dan download file merupakan fitur yang umum digunakan di sebuah website.
Untuk membuat fitur upload, kita sediakan HTML form dengan atribut-atribut sebagai berikut.
<form enctype="multipart/form-data" action="uploader.php" method="post" onsubmit="return checkSize(1048576);">
<input type="file" id="fileupload" name="uploadedfile" /> <input type="submit" value="Upload File" />
Kemudian sediakan field dengan tag input bertipe “file” dan sebuah tombol “Upload File” dengan tag input bertipe ‘submit’. Jika tombol tersebut di click maka akan menjalankan file uploader.php yang ditulis pada form atribut action. Sebelum uploader.php dijalankan, form akan melakukan validasi dengan fungsi javascript checksize(1048576), yakni fungsi javascript untuk membatasi ukuran file yang diupload maksimum 1048576 Bytes atau 1 MB.
Berikut ini coding selengkapnya. Coding ini Anda simpan dengan nama file “index.php”.
Coding dibawah ini berisi form upload dan HTML table yang berisi file yang telah di upload.
Juga link untuk download filenya.
<!doctype html> <html> <head> <title>Upload Download File</title> <style> html, body {font:12px Arial,Helvetica,sans-serif;} fieldset {border:1px solid #ff0000; width:400px;} legend {border:1px solid #ff0000;} table {border-collapse:collapse;width:500px;} td, th {border:1px solid #c0c0c0;padding:5px;} th{background:#ff0000;color:#ffffff;} </style> <script type="text/javascript"> function checkSize(max_img_size) { var input = document.getElementById("fileupload"); if(input.files && input.files.length == 1) { if (input.files[0].size > max_img_size) { alert("Ukuran file harus di bawah " + (max_img_size/1024/1024) + " MB"); return false; } } return true; } </script> </head> <body> <form enctype="multipart/form-data" action="uploader.php" method="post" onsubmit="return checkSize(1048576);"> <fieldset> <legend>Upload File Max 1 MB</legend> Choose a file to upload: <input name="uploadedfile" type="file" id="fileupload" /><br /> <input type="submit" value="Upload File" /> </fieldset> </form> <br/> <table> <tr> <th>File Name</th> <th>Upload Date</th> <th>Type</th> <th>Size</th> <th>Delete</th> </tr> <?php if ($handle = opendir('./files/')) { while (false !== ($file = readdir($handle))) { if($file!=="." && $file !=="..") { echo "<tr><td><a href=\"download.php?id=" . urlencode($file). "\">$file</a></td>"; echo "<td>" . date ("m/d/Y H:i", filemtime("files/".$file)) . '</td>'; echo "<td>" . pathinfo("files/".$file, PATHINFO_EXTENSION) . ' file </td>'; echo "<td>" . round(filesize("files/".$file)/1024) . ' KB</td>'; echo "<td><a href=\"hapus.php?id=$file\">Del</a></td></tr>"; } } closedir($handle); } ?> </table> </body> </html>
Berikut ini coding untuk proses upload file. Coding ini Anda simpan dengan nama file “uploader.php”.
Coding ini akan dijalankan ketika tombol “Upload File” di click.
<?php $target_path = "files/"; $target_path = $target_path . basename( $_FILES['uploadedfile']['name']); if(move_uploaded_file($_FILES['uploadedfile']['tmp_name'], $target_path)) { header("Location: index.php");} else {echo "Error uploading file. Please try again!";} ?>
Coding berikutnya adalah untuk proses download file. Untuk download file yang telah diupload, kita sediakan link di HTML table yang ada di file index.php diatas.
Coding di bawah ini Anda simpan dengan nama file “download.php”.
<?php $direktori = "./files/"; $filename = $_GET['id']; if(file_exists($direktori.$filename)){ $file_extension = strtolower(pathinfo($filename, PATHINFO_EXTENSION)); switch($file_extension){ case "pdf": $ctype="application/pdf"; break; case "exe": $ctype="application/octet-stream"; break; case "zip": $ctype="application/zip"; break; case "rar": $ctype="application/rar"; break; case "doc": $ctype="application/msword"; break; case "xls": $ctype="application/vnd.ms-excel"; break; case "ppt": $ctype="application/vnd.ms-powerpoint"; break; case "gif": $ctype="image/gif"; break; case "png": $ctype="image/png"; break; case "jpeg": case "jpg": $ctype="image/jpg"; break; default: $ctype="application/octet-stream"; } if ($file_extension=='php'){ echo "<h1>Access forbidden!</h1> <p>Please contact Administrator.</p>"; exit; } else{ header("Content-Type: octet/stream"); header("Pragma: private"); header("Expires: 0"); header("Cache-Control: must-revalidate, post-check=0, pre-check=0"); header("Cache-Control: private",false); header("Content-Type: $ctype"); header("Content-Disposition: attachment; filename=\"".basename($filename)."\";" ); header("Content-Transfer-Encoding: binary"); header("Content-Length: ".filesize($direktori.$filename)); readfile("$direktori$filename"); exit(); } }else{ echo "<h1>Access forbidden!</h1> <p>Please contact Administrator.</p>"; exit; } ?>
Coding terakhir ini adalah untuk delete file. Link untuk delete file juga ada di HTML table pada file index.php di atas.
<a href=\"hapus.php?id=$file\">Del</a>
Simpanlah coding di bawah ini dengan nama file “hapus.php”.
<?php $id = $_GET["id"]; unlink("files/".$id); header("location:index.php"); ?>
Silakan Anda coba. Semoga sukses ya…